Show the Outside Temperature
Lua is an interesting Python alternative for small embedded machines that are not sufficiently powerful to run Python scripts. Create Lua socket client/server scripts to send outside temperatures between a Pi and a PS Vita display.
The Lua programming language is often viewed as mainly a video game scripting language, because many popular games such as Angry Birds, Roblox, and World of Warcraft use it. But Lua scripts run extremely fast, making Lua a good option for many small embedded systems where Python isn’t supported or may have performance issues.
In this article, I’ll introduce Lua by letting it fight Python in a simple performance test that compares startup times. Next, I’ll look at some good application fits for Lua, and I’ll finish with a small home project that uses a Raspberry Pi Zero W that sends weather data to a PlayStation (PS) Vita handheld gaming console.
Before jumping into a Lua solution, it’s important to consider the pros and cons. On the positive side, systems like laptops and Raspberry Pis can have Lua initial call-ups 10 times faster than with Python. For very low-end systems with memory constraints, you may see Lua starting more than 30 times faster than Python. On the negative side, Lua doesn’t have the vast libraries, documentation, or user support that is available for Python. For small embedded systems that only require base functionality Lua can be a good fit. However, for large or complex coding projects Python will typically be the better choice.
Lua vs. Python Startup Test
Listing 1 is a Bash script that compares the startup times between Lua and Python.
Listing 1: Test Startup Times on Lua and Python
01 #!/usr/bin/bash
02 # Run timing test for Lua
03 start=$EPOCHREALTIME
04 lua -e "print('Hello from Lua')"
05 end=$EPOCHREALTIME
06 runtime1=$( echo "$end - $start" | bc -l )
07 echo -e "Execution time for Lua: $runtime1 seconds\n"
08
09 # Run timing test for Python3
10 start=$EPOCHREALTIME
11 python3 -c "print('Hello from Python')"
12 end=$EPOCHREALTIME
13 runtime2=$( echo "$end - $start" | bc -l )
14 echo -e "Execution time for Python: $runtime2 seconds\n"
15
16 timediff=$( echo "scale=2; $runtime2/$runtime1" | bc -l )
17 echo "Lua starts $timediff times faster than Python"
Startup times will vary based on hardware, available memory, and background CPU activity. For laptops and Raspberry Pi 4 modules, Lua tends to be up to 10 times faster than Python. On lower-end platforms like a Raspberry Pi B+ or an Arduino Yún, both running OpenWrt, Lua can be 30 times faster.
Fast initial startup is especially useful for web pages. I’ve created a Bash script that checks whether Lua is a worthwhile fit for your project’s hardware (see Listing 1). For longer running applications, Lua performance can be further enhanced by using LuaJIT , a Lua just-in-time (JIT) compiler.
OpenWrt and Lua
OpenWrt is a networking-focused operating system that runs on over 2,000 different embedded devices. OpenWrt is most commonly used on routers, but it can also revitalize many older pieces of equipment thanks to its extremely lean design. For example, you can load OpenWrt on the original Raspberry Pi B+ or lower-end Pi Zero W modules, and they’ll perform astonishingly well. See the OpenWrt installation pages for instructions and download files for your specific hardware.
OpenWrt does not provide a desktop GUI; instead, it uses a web interface that is based on Lua and the LuCI framework. You can install Python on OpenWrt systems, but due to Lua’s speed and the fact that it’s preinstalled, Lua is an option that should be considered. Lua can also be useful for connecting command-line tools or as an alternative to Bash scripts.
There are many hardware applications where Lua can be used to monitor or change device settings. Listing 2 is a Lua CGI web script that shows USB devices and power settings, and it toggles the power on USB port 3. This example uses the output from two command-line tools: lsusb
lists the USB devices, and uhubctl
lets you view and toggle USB power.
Listing 2: Show USB Devices and Power
01 #!/usr/bin/lua
02 -- showusb.lua - show USB devices and power, then toggle power on port 3
03 print("Content-type: text/html; charset=utf-8\n\n")
04 print("<h1>OpenWrt/Lua - USB Example</h1><hr>")
05
06 -- use lsusb to show USB devices
07 f = assert(io.popen("lsusb"))
08 print("<h2>USB Device Info</h2>")
09 print("<pre>" .. assert(f:read('*a')) .. "</pre><hr>" )
10
11 -- toggle power on USB port 3 then show status
12 f = assert(io.popen("uhubctl -l 1-1 -p 3 -a toggle"))
13 f = assert(io.popen("uhubctl | grep 'hub 1-1' -A4"))
14 print("<h2>USB Port Power Status</h2>")
15 print("<pre>" .. assert(f:read('*a')) .. "</pre><hr>" )
Lua runs external programs through a combination of an
assert(io.popen("cmd"))
function call (which runs a command cmd with error recovery enabled) and a
read('*a')
read-all call that retrieves the output of the command cmd
.
Custom web pages can use OpenWrt’s web server by adding files to the /www/cgi-bin
directory. Figure 1 shows a Raspberry Pi 4 with a USB light being toggled by the Lua web page.
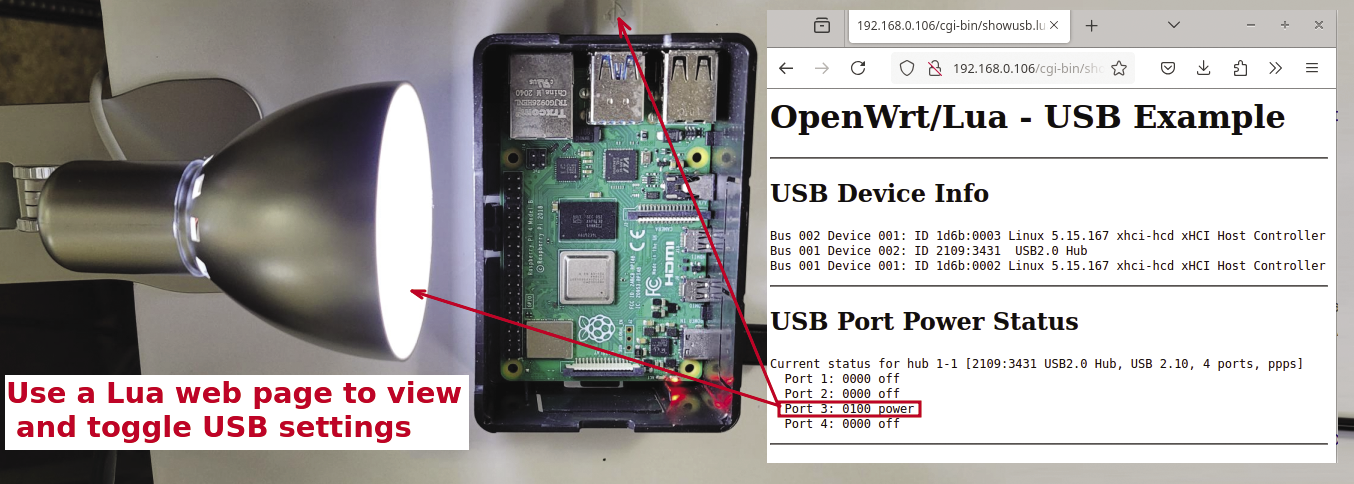
Handheld Gaming Consoles
Most of the older Sony and Nintendo handheld gaming consoles support Lua, so you can use the original or today’s retro handheld units as Internet of Things (IoT) monitoring devices. Some of these handhelds support a stackless version of Python 2.x. While these Python ports were often excellent proofs of concept, they unfortunately lack documentation or user examples. The Lua ports for the handheld world have many full-featured offerings that include documentation, samples, and user-created games.
Figure 2 shows two popular gaming handhelds, the Nintendo DS and the Sony PlayStation Portable (PSP), both of which support WiFi and Lua programming. Unfortunately, you cannot get WPA2 WiFi security with them since they are too old, but they do support WEP and open connections. (Note: the new retro gaming clones available on the market will probably support WPA2). The latest Nintendo handheld, the Switch, does not support Homebrew applications, so custom Python or Lua coding is not possible. However, you can buy FUZE4 Nintendo Switch, a coding application, in the My Nintendo Store.
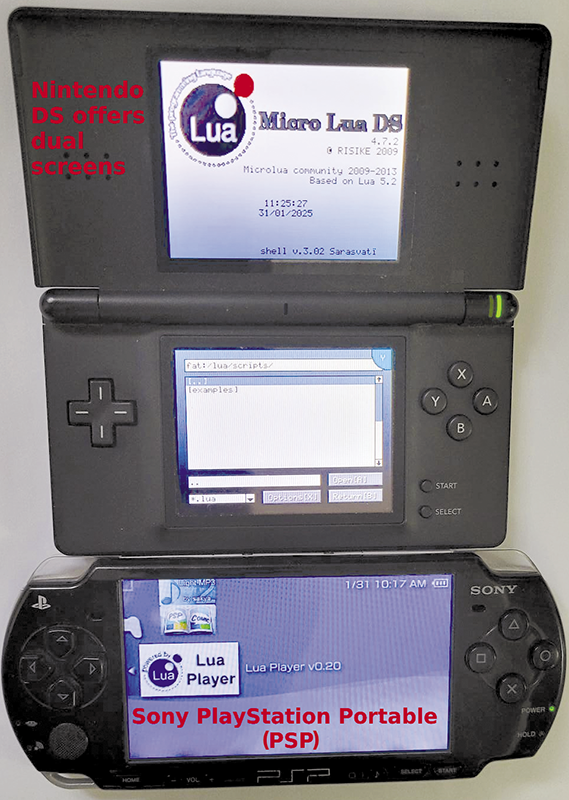
Raspberry Pi + PS Vita Example
For this article, I put a Raspberry Pi Zero W with a BME280 atmospheric sensor in a plastic box (Figure 3). I was probably pushing the specs on the Pi Zero W, because we had some temperatures below -20°C (-4°F).
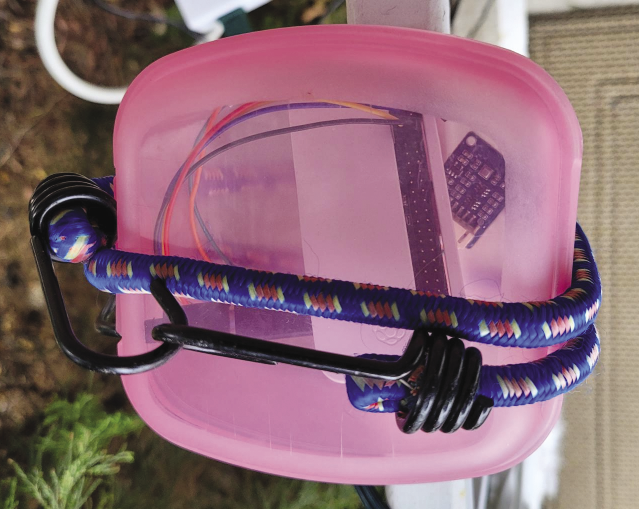
Lua presently doesn’t have a native BME280 sensor library module, so I downloaded an example in C and used the temperature value as the message sent from the Pi’s TCP socket server script (Listing 3). Note that this script (outside.lua
) needs access to network ports so you have to run it with sudo
.
Listing 3: Sending the BME280 Temperature Value
01 #!/usr/bin/lua
02 ‑‑ outside.lua
03 local socket = require("socket")
04 ‑‑ create a TCP socket and bind it to the local host, on port 100
05 local server = assert(socket.bind("*", 100))
06 ‑‑ find out which port the OS chose for us
07 local ip, port = server:getsockname()
08 print ("Starting TCP socket server on: " .. ip .. " port: " .. port)
09
10 ‑‑ loop forever waiting for clients
11 while 1 do
12 ‑‑ wait for a connection from any client
13 local client = server:accept()
14 client:settimeout(2)
15
16 ‑‑ receive the line and print the message
17 local line, err = client:receive()
18 print(line)
19
20 ‑‑ send the temperature to the socket client
21 f = assert(io.popen("/home/pete/outside/bme280"))
22 result = assert(f:read('*a'))
23 msg = result .. " C\n"
24 print(msg)
25 if not err then client:send(msg) end
26 ‑‑ done with client, close the object
27 client:close()
28 end
There are many different WiFi enabled gaming consoles that could be used – I picked a Sony PS Vita that supports WPA wireless networking. Homebrew applications can be installed on the PS Vita using the VitaDB Downloader tool, and the VitaShell utility lets you access the PS Vita’s filesystem via USB or FTP connections (Figure 4). The Lua Player Plus Vita repository includes about 20 code samples; one of them is a socket client. I modified that socket example to include larger fonts and a timer component. To run a custom Lua simply add it to the ux0:data/lpp-vita
directory. Figure 5 shows the game console displaying the outside temperature as measured by the Raspberry Pi.
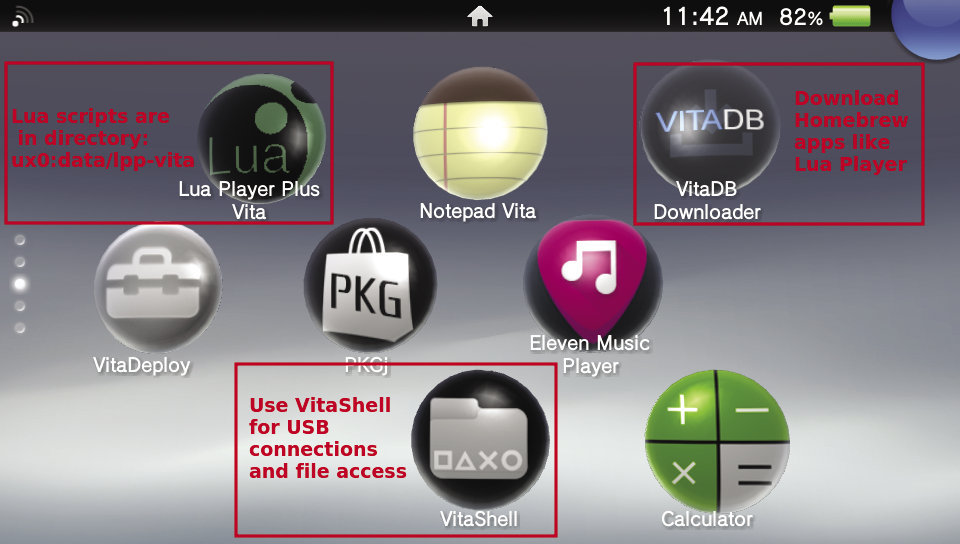
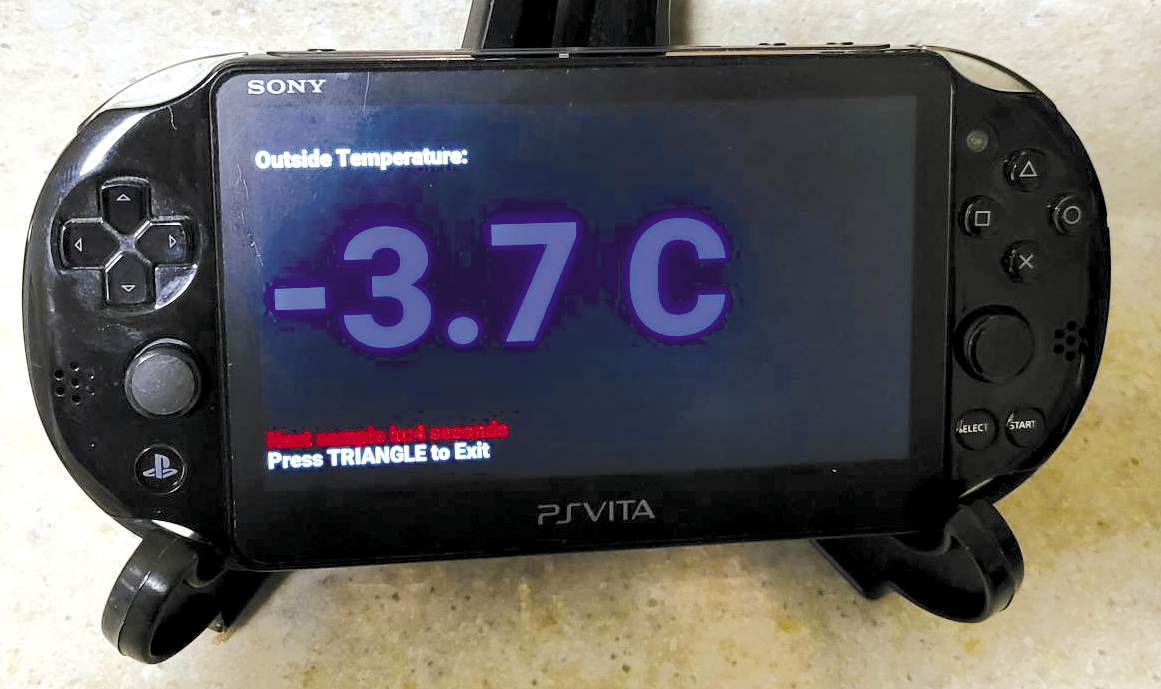
Summary
As always, it’s important to know which options are available and their specific benefits and drawbacks. Lua will run much faster than an equivalent Python script, with the biggest noticeable difference in startup times. However, there are vast collections of libraries and user documentation for Python. Some areas that might benefit from using Lua are:
- Replacing Bash scripts: Bash has poor support for floating-point numbers, and the Bash syntax can be quite difficult to read. Lua can be a good alternative for creating system tools.
- Networking tools: Because of Lua’s lightweight design, it could be a good choice for messaging queue (AMQP or MQTT), Modbus, or socket server interfaces. It’s worth noting that Lua is the script language used in Redis and the NMAP Scripting Engine (NSE).
- Web pages: Lua’s fast initial startup times make it an excellent option for user interfaces.
- Game consoles: You can use Lua to repurpose your old gaming handheld into an IoT monitoring device.