Cooking Pi
Create GUIs and a web app that connects to sensors.
Julia is a relatively new general-purpose programming language that has an emphasis on data science and artificial intelligence (AI). In the past few years, Julia has been gaining momentum. Like Python and R, Julia can be used in Jupyter notebooks.
A Raspberry Pi is a great platform for an introduction to Julia programming, and Python users will be happy to hear that Python code can be integrated directly into Julia scripts. In this article, I look at creating a Julia graphical user interface (GUI), micro-web server, and charting apps that communicate with Raspberry Pi hardware. I’ll also introduce mixing Python code within Julia script to communicate with an LCD display.
Getting Started
Julia is supported on Windows, macOS, and Linux. You can find the download instructions for your specific system online. The latest supported version of Julia can be installed on a Raspberry Pi with the Snap package manager. To install Snap, enter
sudo apt update
sudo apt install snapd
sudo reboot
After the Raspberry Pi has restarted, install Julia with
sudo snap install core
sudo snap install julia --classic
The command
sudo apt install julia
also installs Julia; however, you will get an older version. To check the version of Julia, use either the -v
option (Figure 1) or simply run julia
.
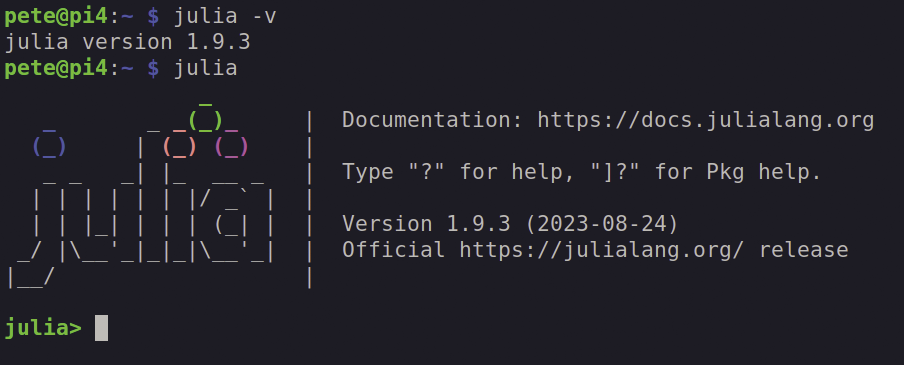
Your project setup will vary by the sensors and hardware you have available. For my setup I used a 16x2 LCD screen and a BME280 temperature-humidity-pressure sensor, both wired into the Raspberry Pi through the inter-integrated circuit (I2C) connection. To simplify the wiring, the hardware was connected by a Pimoroni Explorer HAT (~$25), which has four built-in colored LEDs and a small prototyping breadboard (Figure 2).
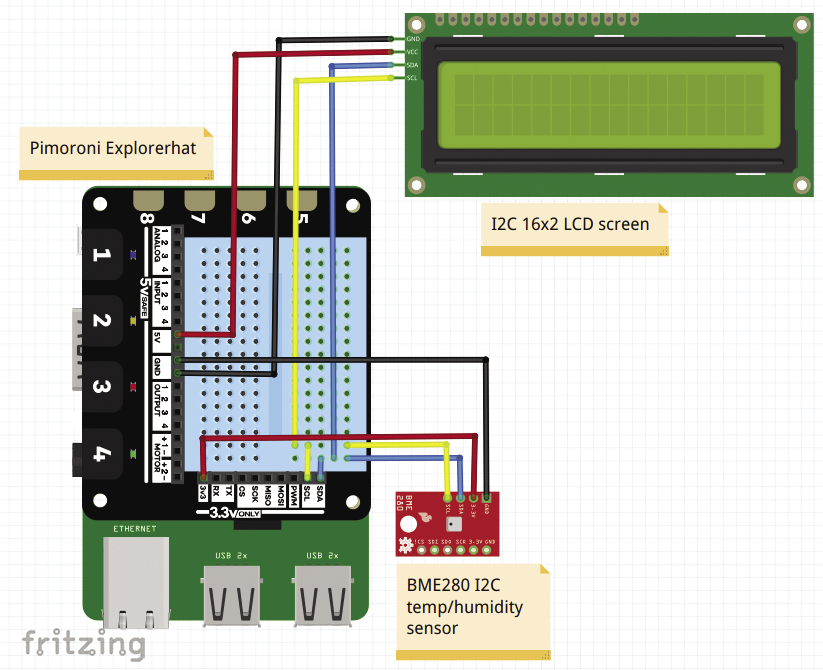
The I2C protocol uses a multimain-multisub serial communication bus, with the serial data line (SDA) on Raspberry Pi pin 3 and the serial clock line (SCL) on pin 5.
Julia with Raspberry Pi GPIO
Communicating with the Raspberry Pi’s general-purpose input/output (GPIO) pins uses Julia’s PiGPIO package, which can be installed at the command line:
$ julia --eval 'using Pkg; Pkg.add("PiGPIO")'
To install and view the status of packages within Julia, use
julia> # load the Pkg module
julia> using Pkg
julia> # install a new package
julia> Pkg.add("PiGPIO")
julia> # view the status of installed packages
julia> Pkg.status()
Status `~/.julia/environments/v1.9/Project.toml`
[bb151fc1] PiGPIO v0.2.0
Once this package is installed, the pigpiod
daemon is created, to which the Julia script connects. The pigpiod
daemon needs to be started before Julia can connect to the Raspberry Pi pins:
sudo pigpiod
To load the PiGPIO library, create the Pi object (p
), set the mode of the pin, and finally write to and read from GPIO pin 17, use the interactive code
julia> using PiGPIO;
julia> ledpin = 17;
julia> p = Pi();
julia> set_mode(p,ledpin, PiGPIO.OUTPUT);
julia> PiGPIO.write(p, ledpin, PiGPIO.HIGH);
julia> PiGPIO.read(p, ledpin)
1
Note that adding a semicolon at the end of a line suppresses debug information. To use a read
interactively, ensure that you do not use the semicolon.
Now that the basic communications are working between Julia and the Raspberry Pi pins, you can create GUI and web server apps.
GUIs with GTK
Like most programming languages, Julia has a number of GUI options. For my testing I used the GTK library, which is installed in Julia with
julia> using Pkg
julia> Pkg.add("Gtk")
Listing 1 is a Julia script to create a two-button window that will turn on or turn off a GPIO pin. The Julia syntax looks somewhat like Python or Matlab. Libraries are imported by the using
command (lines 4-5). Functions are defined with a function
statement and closed with an end
(lines 32-35 and 38-41). A do
block (lines 45-47) groups logic with a function.
Listing 1: Toggle a GPIO Pin
01 #
02 # Gtk_17.jl - Use a Gtk GUI to toggle a PI GPIO LED
03 #
04 using Gtk;
05 using PiGPIO;
06
07 # Setup GPIO
08 p=Pi();
09 led_pin = 17;
10 set_mode(p, led_pin, PiGPIO.OUTPUT)
11
12 # Init GUI objects
13 win = GtkWindow("Toggle a Pi LED", 300, 300);
14 g = GtkGrid();
15
16 # Text label
17 label = GtkLabel(string("Pin : $led_pin"));
18 # Buttons
19 btn_on = GtkButton("ON");
20 btn_off = GtkButton("OFF");
21
22 # Put GUI widgets into a grid
23 g[1:2] = label; # Cartesian coordinates, g[x,y]
24 g[1,2] = btn_on;
25 g[2,2] = btn_off;
26
27 # Show the GUI Window
28 push!(win, g);
29 showall(win);
30
31 # Callbacks
32 function turn_on(w1)
33 PiGPIO.write(p, led_pin, PiGPIO.HIGH)
34 println("ON button pressed")
35 end
36 signal_connect(turn_on, btn_on, "clicked")
37
38 function turn_off(w2)
39 PiGPIO.write(p, led_pin, PiGPIO.LOW)
40 println("OFF button pressed")
41 end
42 signal_connect(turn_off, btn_off, "clicked")
43
44 # Close cleanly
45 signal_connect(win, :destroy) do widget
46 Gtk.gtk_quit()
47 end
48 Gtk.gtk_main()
This Julia script is run by
$ julia gtk_17.jl
Figure 3 shows the Julia GTK GUI window toggling GPIO pin 17 (the yellow LED 2 on the Pi Explorer HAT).
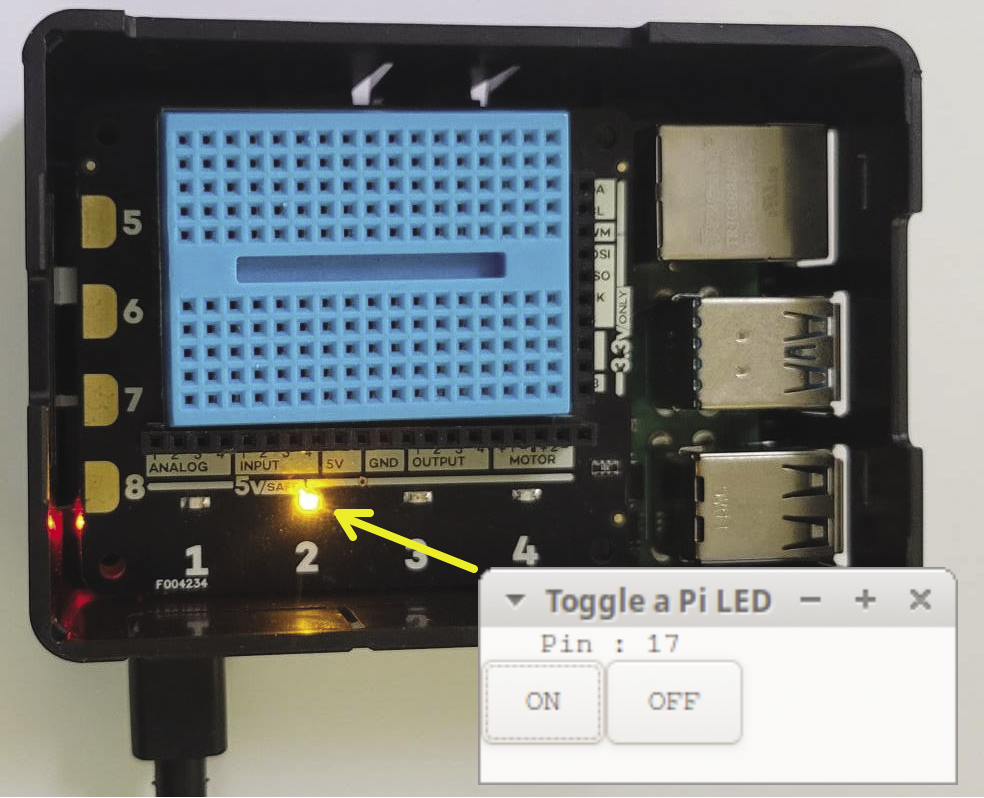
Julia Web Server
As with graphics libraries, you have a few web server options. I found that the Genie web framework was one of the easiest to get up and running. Listing 2 is an example web app that creates two buttons to turn a GPIO pin on and off (Figure 4).
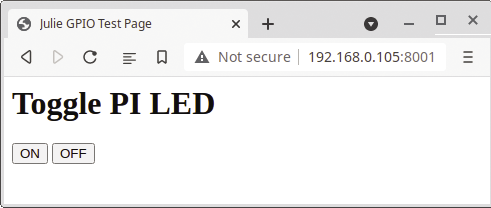
Listing 2: Genie Web Framework
01 #
02 # web2gpio.jl - Julia Web Page with routes to turn on/off a Pi GPIO pin
03 #
04 using Genie
05 using Sockets # to get IP address
06 using PiGPIO;
07
08 # Setup GPIO
09 p=Pi();
10 led_pin = 17;
11 set_mode(p, led_pin, PiGPIO.OUTPUT)
12
13 # get the Rasp Pi IP address
14 myip = string(getipaddr() )
15
16 println("Loading Genie...")
17
18 # create an HTML document
19 html_file = """
20 <html>
21 <head>
22 <title>Julie GPIO Test Page</title>
23 </head>
24 <body>
25 <h1>Toggle Pi LED</h1>
26 <form>
27 <button> type="submit" formaction="/ON">ON</button>
28 <button type="submit" formaction="/OFF">OFF</button>
29 </form>
30 </body>
31 </html>
32 """
33
34 route("/") do
35 return html_file
36 println("Connected")
37 end
38
39 route("/ON?") do
40 PiGPIO.write(p, led_pin, PiGPIO.HIGH)
41 return html_file
42 println("ON Selected")
43 end
44
45 route("/OFF?") do
46 PiGPIO.write(p, led_pin, PiGPIO.LOW)
47 return html_file
48 println("OFF Selected")
49 end
50
51 # Start the web app on port 8001
52 up( 8001, myip, async = false)
This script uses three libraries: Genie for the web server framework, Sockets to get the Raspberry Pi’s IP address, and PiGPIO to connect to the GPIO pins (lines 4-6). Pin 17 is set as an output in lines 9-11. To keep things simple, the HTML code is defined as a variable (lines 19-32). For larger projects I would recommend the use of external HTML files.
The Julia code defines three routes (lines 34-49). The first route is the default (/) page that prints out the html_file
variable. The /ON
and /OFF
routes set the GPIO pin output when either the ON or OFF form buttons are pressed.
The final line in the script sets up the web framework to run on port 8001 with the Raspberry Pi’s IP address.
The command
julia web2gpio.jl
starts the Julia web script.
Plot BME280 Sensor Data
For the final example, the goal is to create a dynamic bar chart that shows BME280 temperature and humidity data. The Plots charting library is installed by
julia> using Pkg
julia> Pkg.add("Plots")
Unfortunately, unlike Python and Node-RED, Julia does not have Raspberry Pi sensor libraries, so for this project a BME280 Bash command-line utility is used:
$ # Install a Python based Bash command line tool
$ sudo python -m pip install bme280
BME280 sensors typically use the I2C address 0x76 or 0x77. The i2cdetect
tool can be used to identify your sensor address by entering
i2cdetect -y 1
Within Julia, external command strings are defined with backticks (`). This example creates a command variable (mycmd), checks the variable type, and runs the command
julia> mycmd = `pwd`; # pwd=present working directory
julia> typeof(mycmd)
Cmd
julia> run(mycmd) ; # run the command
/home/pete/
The Julia read
function runs an external command and stores the output string as a variable (Figure 5). Next, a split
creates an array of string output from the BME280 data. For this example, the humidity value is the third index (data[3]
), and temperature is the fifth index (data[5]
).
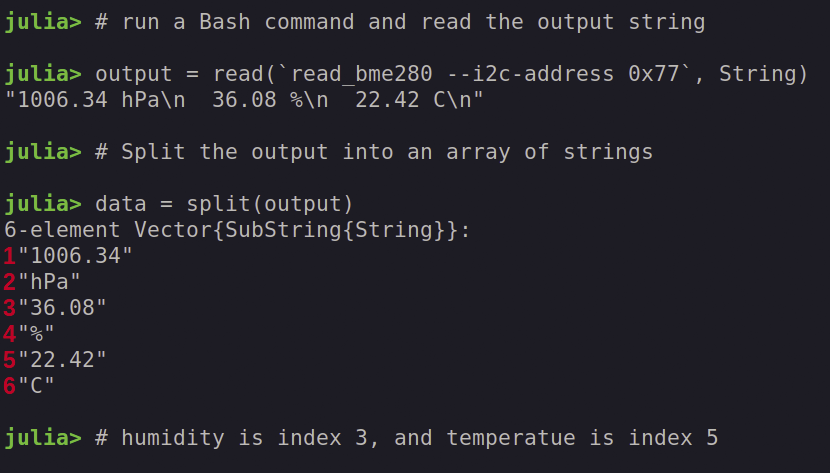
Once the BME280 humidity and temperature values can be extracted, the next step is to write a Julia script that periodically scans the sensor and passes the values to a bar chart.
Listing 3 uses a while
loop that cycles every 10 seconds (lines 9-28), and as in the previous example, the sensor data is read from the Bash command and then passed to a data array (lines 12 and 13). The temperature and humidity values are parsed as floats (lines 16 and 19) and shown in a bar chart (lines 23-25).
Listing 3: Plot BME280 Sensor Data
01 # !/snap/bin/julia
02 #
03 # bme_280.jl - show BME280 sensor data on a refreshing bar chart
04 #
05 using Plots
06 using Dates
07
08 print("Show BME280 readings every 10 seconds. Control-C to exit.\n")
09 while true
10
11 # get the sensor output, then split it into an array of strings
12 output = read(`read_bme280 --i2c-address 0x77`, String)
13 data = split(output)
14
15 # get the temperature as a Float, (5th item in array)
16 t = parse(Float64,data[5])
17
18 # get the humidity as a Float, (3rd item in array)
19 h = parse(Float64,data[3])
20
21 # define a bar chart
22 thetitle = "BME280 Data - " * Dates.format(now(), "H:MM:SS")
23 p = bar(["Temperature (C)","Humidity (%)"], [t,h],legend=false,
24 title=thetitle, texts = [t,h], color=[:red, :yellow] )
25 display(p)
26
27 sleep(10)
28 end
The following script has the location of the Julia binary in the first line, so the file can be run like a Bash or Python application:
$ # enable the execute status on the file
$ sudo chmod +x bme_280.jl
$ # run the script directly
$ ./bme_280.jl
Figure 6 shows the BME280 bar chart app with the Raspberry Pi hardware. A nice enhancement to this project would be to save the sensor data to a database or file and then present the results in a moving real-time line chart.
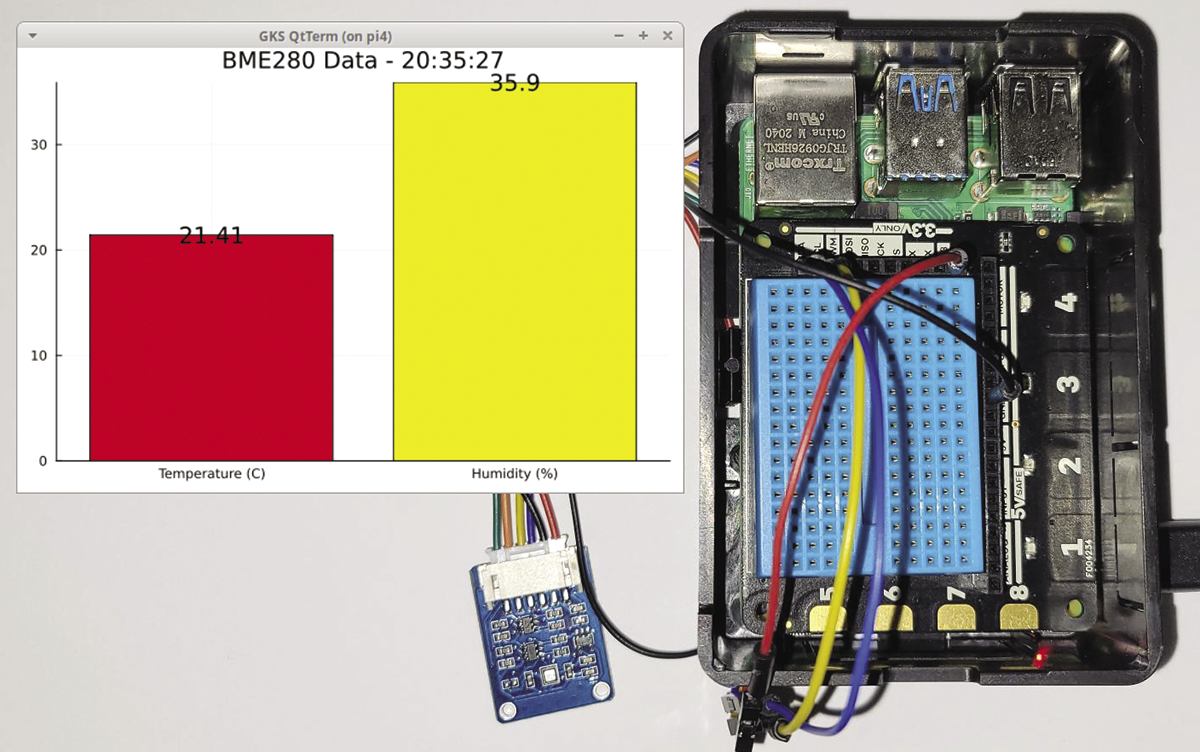
Julia Calling Python
One of the interesting features in Julia is that script languages such as Lua, Matlab, R, and Python can be integrated directly into Julia code.
To enable this functionality, the first step is to install the required language library. To add the Python interface library, enter
$ julia --eval 'using Pkg; Pkg.add("PyCall")'
Most of the Raspberry Pi sensors and third-party devices are supported in Python, so the PyCall library can come in extremely handy when you want to communication with any external Pi hardware.
A simple example uses PyCall
with a 16x2 LCD display. The first step in the integration is to install Python’s Pi LCD library:
$ # install the Python Raspberry Pi LCD library
$ pip install rpi-lcd
PyCall
works in a couple of different ways. The first is Python code used directly within a Julia script, and the second is to have Julia code reference Python objects. An example runs Python code directly to write text to a two-line I2C LED screen:
julia> using PyCall
julia> py"""
# Use Python to write text to a 2-line LCD screen
from rpi_lcd import LCD
lcd = LCD()
lcd.text('Hello World!', 1)
lcd.text('Raspberry Pi', 2)
"""
julia> # Now back into Julia
The py
statement runs a string of multiple Python lines. This way can be a little tricky because errors occur if the string does not adhere to Python’s indentation rules.
The same functionality with
using PyCall
rpi_lcd = pyimport("rpi_lcd");
lcd = rpi_lcd.LCD();
lcd.text("Hello from Julia", 1)
lcd.text("Raspberry Pi!", 2)
is achieved by referencing a Python object in Julia (Figure 7).
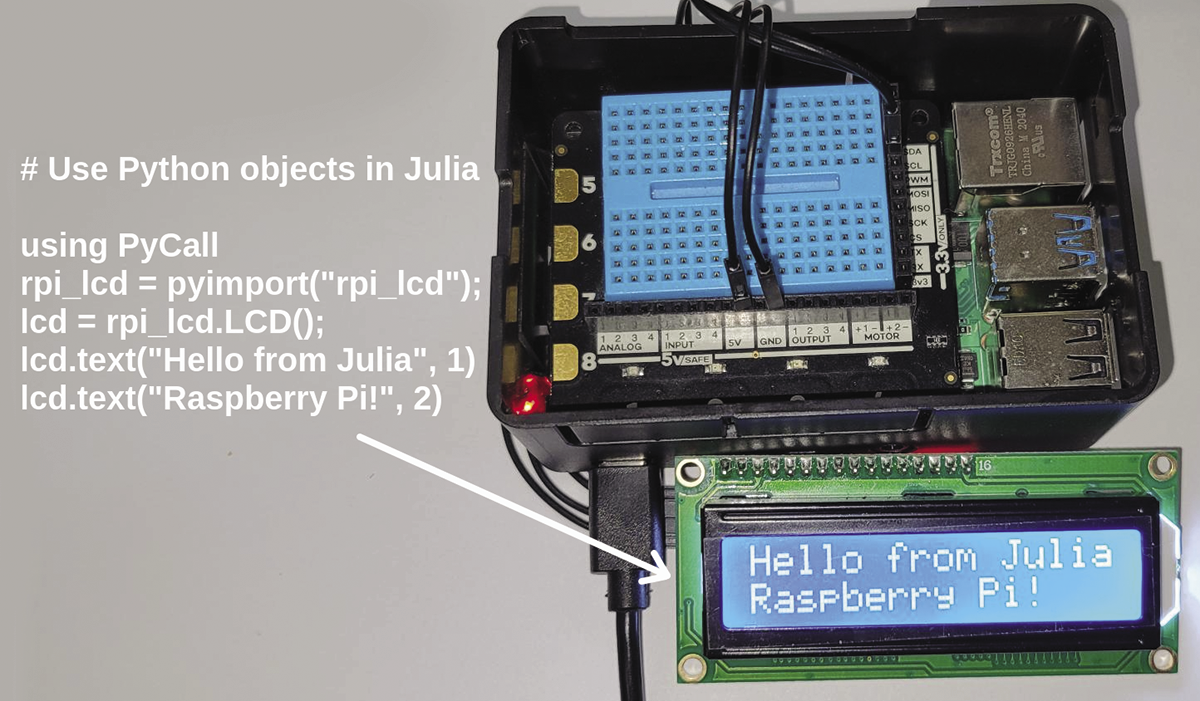
Summary
A Raspberry Pi can be a nice platform to use to get to know Julia programming. For Python users, the mixing of Python code into Julia scripts allows common Pi hardware to be integrated into your projects.
After getting a handle on some of the Julia basics, the next steps might be to investigate data analysis, Jupyter notebooks, or AI applications.
It should be noted that playing with Julia on older Raspberry Pi hardware or using early versions of Julia could run painfully slow. If speed becomes an issue, Julia offers a number of packaging and pre-compilation options to improve call-up time.